<script src="https://unpkg.com/react@17/umd/react.development.js"></script>
<script src="https://unpkg.com/react-dom@17/umd/react-dom.development.js"></script>
<script src="https://unpkg.com/babel-standalone@6/babel.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jspdf/2.5.1/jspdf.umd.min.js"></script>
<script type="text/babel">
const { jsPDF } = window.jspdf;
const PropertyInspectionChecklist = () => {
const [formData, setFormData] = React.useState({
propertyAddress: "",
inspectorName: "",
inspectionDate: new Date().toISOString().split("T")[0],
conditionOptions: [
{ value: "Good", label: "Good" },
{ value: "Needs Repair", label: "Needs Repair" },
{ value: "Hazardous", label: "Hazardous" },
],
sections: [
{
title: "Exterior",
items: [
{ label: "Roof Condition", conditionField: "roofCondition", commentField: "roofComments" },
{ label: "Walls Condition", conditionField: "wallsCondition", commentField: "wallsComments" },
],
},
{
title: "Interior",
items: [
{ label: "Living Room", conditionField: "livingRoomCondition", commentField: "livingRoomComments" },
{ label: "Kitchen", conditionField: "kitchenCondition", commentField: "kitchenComments" },
],
},
],
});
const handleConditionChange = (sectionIndex, itemIndex, value) => {
const updatedSections = [...formData.sections];
const conditionField = updatedSections[sectionIndex].items[itemIndex].conditionField;
setFormData((prev) => ({
...prev,
[conditionField]: value,
}));
};
const handleCommentChange = (sectionIndex, itemIndex, value) => {
const updatedSections = [...formData.sections];
const commentField = updatedSections[sectionIndex].items[itemIndex].commentField;
setFormData((prev) => ({
...prev,
[commentField]: value,
}));
};
const generatePDFReport = () => {
const doc = new jsPDF();
doc.setFontSize(18);
doc.setTextColor(0, 51, 102);
doc.text("Property Inspection Report", 105, 20, null, null, "center");
doc.setFontSize(12);
doc.text(`Property Address: ${formData.propertyAddress || "Not Specified"}`, 20, 35);
doc.text(`Inspector Name: ${formData.inspectorName || "Not Specified"}`, 20, 45);
doc.text(`Inspection Date: ${formData.inspectionDate}`, 20, 55);
let yPosition = 70;
formData.sections.forEach((section) => {
doc.setFontSize(14);
doc.setTextColor(0, 51, 102);
doc.text(section.title, 20, yPosition);
yPosition += 10;
section.items.forEach((item) => {
const condition = formData[item.conditionField];
const comments = formData[item.commentField];
if (condition) {
doc.text(`${item.label}: ${condition}`, 20, yPosition);
yPosition += 7;
if (comments) {
const splitComments = doc.splitTextToSize(comments, 170);
doc.text(splitComments, 30, yPosition);
yPosition += splitComments.length * 5 + 5;
}
yPosition += 5;
}
if (yPosition > 280) {
doc.addPage();
yPosition = 20;
}
});
yPosition += 10;
});
const conditions = formData.sections.flatMap((section) =>
section.items.map((item) => formData[item.conditionField])
).filter(Boolean);
const overallCondition = conditions.every((c) => c === "Good")
? "Good"
: conditions.some((c) => c === "Hazardous")
? "Hazardous"
: "Needs Repair";
doc.setFontSize(12);
doc.text(`Overall Condition: ${overallCondition}`, 20, yPosition);
doc.save(`Inspection_Report_${formData.inspectionDate}.pdf`);
};
return (
<div className="max-w-6xl mx-auto p-6 bg-gray-50">
<h1 className="text-3xl font-bold text-center mb-6 text-blue-900">
Property Inspection Checklist
</h1>
<div className="bg-white shadow-md rounded-lg p-6 mb-6">
<h2 className="text-2xl font-bold text-blue-800 mb-4">Property Details</h2>
<div className="grid grid-cols-1 md:grid-cols-2 gap-4">
<div>
<label className="block mb-2 font-bold">Property Address</label>
<input
type="text"
className="w-full p-2 border rounded"
value={formData.propertyAddress}
onChange={(e) =>
setFormData((prev) => ({ ...prev, propertyAddress: e.target.value }))
}
placeholder="Enter property address"
/>
</div>
<div>
<label className="block mb-2 font-bold">Inspector Name</label>
<input
type="text"
className="w-full p-2 border rounded"
value={formData.inspectorName}
onChange={(e) =>
setFormData((prev) => ({ ...prev, inspectorName: e.target.value }))
}
placeholder="Enter inspector name"
/>
</div>
</div>
</div>
{formData.sections.map((section, sectionIndex) => (
<div key={section.title} className="bg-white shadow-md rounded-lg p-6 mb-6">
<h2 className="text-2xl font-bold text-blue-800 mb-4">{section.title}</h2>
<div className="space-y-4">
{section.items.map((item, itemIndex) => (
<div key={item.label} className="grid grid-cols-1 md:grid-cols-3 gap-4">
<div>
<label className="block mb-2 font-bold">{item.label}</label>
</div>
<div>
<select
className="w-full p-2 border rounded"
value={formData[item.conditionField] || ""}
onChange={(e) =>
handleConditionChange(sectionIndex, itemIndex, e.target.value)
}
>
<option value="">Select Condition</option>
{formData.conditionOptions.map((option) => (
<option key={option.value} value={option.value}>
{option.label}
</option>
))}
</select>
</div>
<div>
<textarea
className="w-full p-2 border rounded"
placeholder="Additional comments"
value={formData[item.commentField] || ""}
onChange={(e) =>
handleCommentChange(sectionIndex, itemIndex, e.target.value)
}
rows="2"
/>
</div>
</div>
))}
</div>
</div>
))}
<div className="text-center mt-6">
<button
className="bg-blue-800 text-white px-6 py-3 rounded-lg hover:bg-blue-700 transition-colors"
onClick={generatePDFReport}
>
Generate Inspection Report PDF
</button>
</div>
</div>
);
};
ReactDOM.render(
<React.StrictMode>
<PropertyInspectionChecklist />
</React.StrictMode>,
document.getElementById("root")
);
</script>

St. Mary's Real Estate
512 W Bertrand Ave
St Marys, KS 66536
Joseph Kirby
Broker
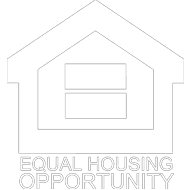
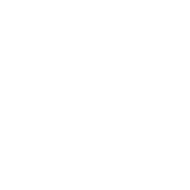